반응형
1) File - 두 가지로 분류 (Internal Storage, External Storage)
2) Shared Preference
3) Data base
4) Web서버
이전까지는 메모장에 저장하는 방법이었다면, 만약 회원 정보를 기록을 한다면 엑셀 같은 것을 사용할 것이다.
Data base는 자료 표현하는 단위, 그리고 프로그램(MySQL, MsSQL)이 있다.
Data base를 사용하려면 프로그램이 필요하다. 안드로이 운영체제를 사용하면 자동으로 SQLite가 있다.(핸드폰에)
이번 예제는 내 핸드폰 안에 저장을 할 것이다. Data.db 안에 member 표(table)를 만들것이다.
( 다른 휴대폰에서는 데이터를 볼 수 없다. 추후에 서버를 이용한 Data base를 해보겠다.)
<실행 화면>
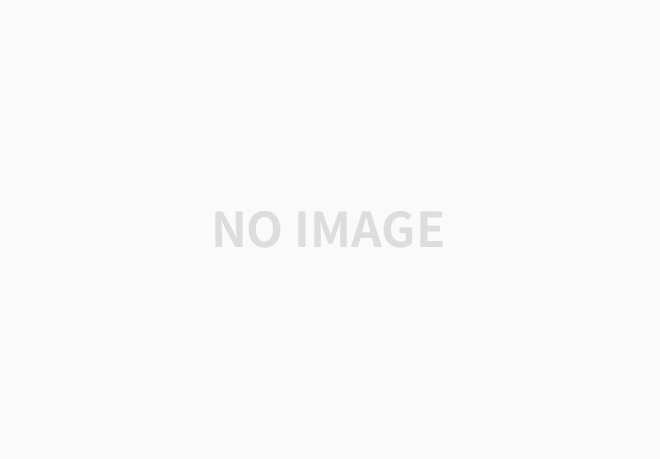
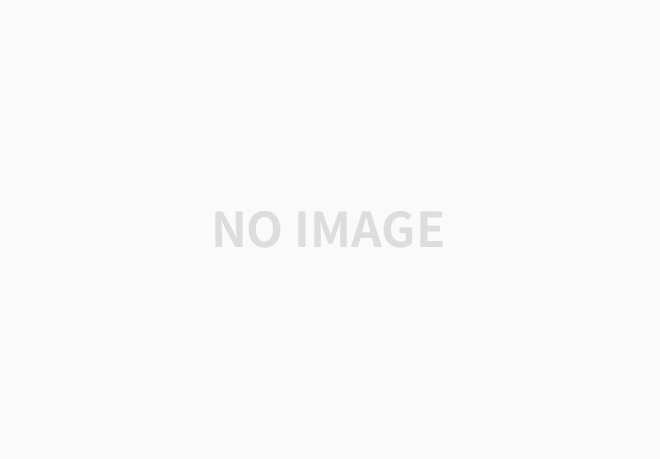
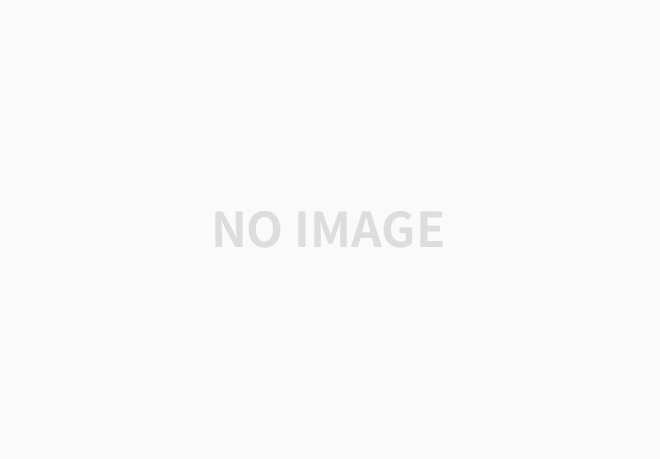
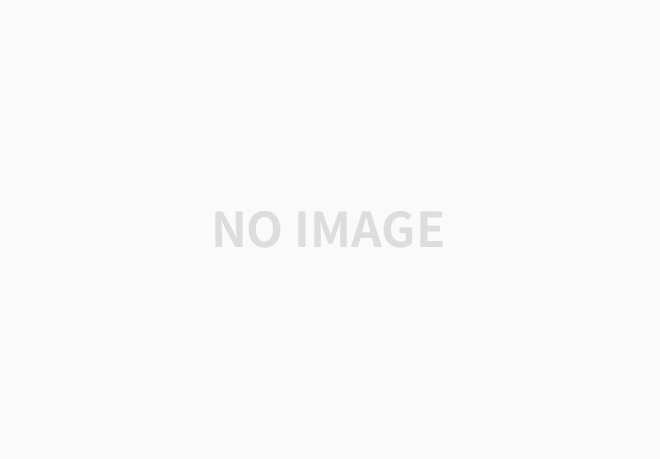
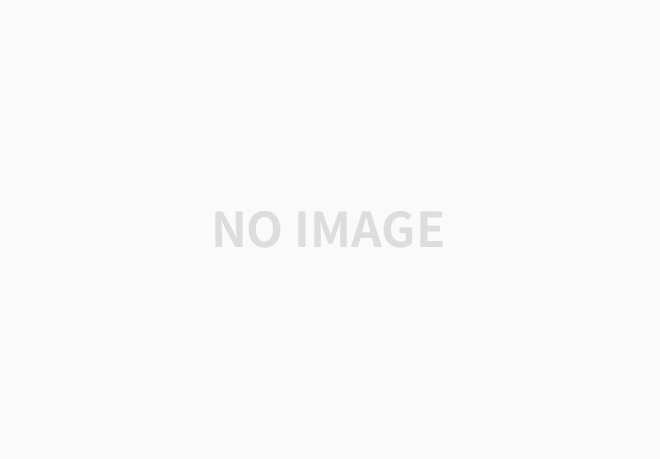
저장 경로
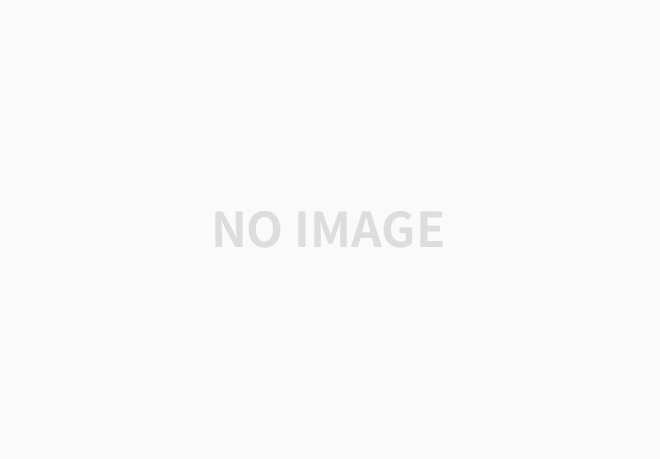
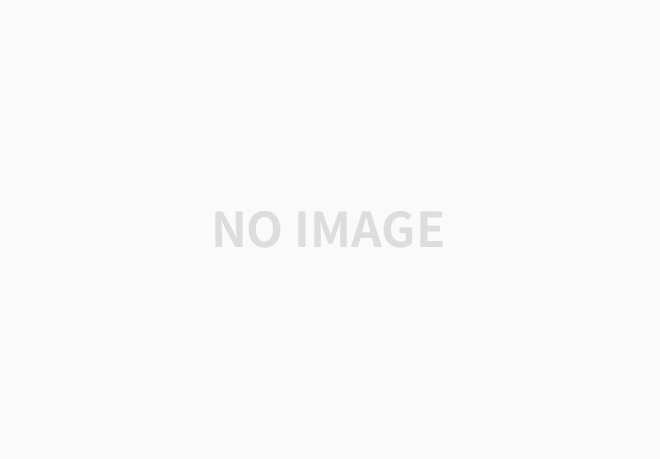
3) Data base
activity_main.xml 코드
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
|
<?xml version="1.0" encoding="utf-8"?>
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp"
tools:context=".MainActivity">
<EditText
android:id="@+id/et_name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="input name"
android:inputType="text"/>
<EditText
android:id="@+id/et_age"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="input age"
android:inputType="number"/>
<EditText
android:id="@+id/et_email"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="input email"
android:inputType="textEmailAddress"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="insert"
android:onClick="clickInsert"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="select all"
android:onClick="clickSelectAll"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="select by name"
android:onClick="clickSelectByName"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="update by name"
android:onClick="clickUpdate"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="delete by name"
android:onClick="clickDelete"/>
</LinearLayout>
|
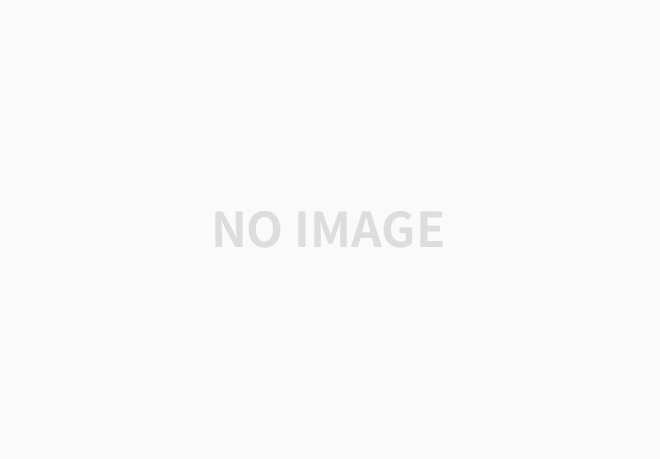
MainActivity.java 코드
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
|
import android.app.AlertDialog;
import android.database.Cursor;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
EditText etName, etAge, etEmail;
String tableName="member"; // 표 이름
SQLiteDatabase db;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
etName=findViewById(R.id.et_name);
etAge=findViewById(R.id.et_age);
etEmail=findViewById(R.id.et_email);
//dbName으로 데이터베이스 파일 생성 또는 열기
db= this.openOrCreateDatabase(dbName, MODE_PRIVATE, null); //db문서를 열거나, 없으면 만드는 작업
//만들어진 DB파일에 "member"라는 이름으로 테이블 생성 작업 수행
db.execSQL("CREATE TABLE IF NOT EXISTS "+tableName+"(num integer primary key autoincrement, name text not null, age integer, email text);");//괄호 안에 SQL언어 쓰면 됨.
//IF NOT EXISTS <- 만약 존재하지 않으면 만들자.
}
public void clickInsert(View view) {
String name= etName.getText().toString();
int age=Integer.parseInt(etAge.getText().toString());
String email=etEmail.getText().toString();
//데이터베이스에 데이터를 삽입(insert)하는 쿼리문 실행
db.execSQL("INSERT INTO "+tableName+"(name, age, email) VALUES('"+name+"','"+age+"','"+email+"');");
etName.setText("");
etAge.setText("");
etEmail.setText("");
}
public void clickSelectAll(View view) {
//*은 모든 데이터가 아닌 모든 컬룸을 말하고 두번째 파라미터가 null이기 때문에 모든 데이터를 가져온다.
Cursor cursor =db.rawQuery("SELECT * FROM "+tableName+"",null); //두 번째 파라미터: 겁색 조건
//Cursor객체: 결과 table을 참조하는 객체
//Cursor객체를 통해 결과 table의 값들을
//읽어오는 것임.
if(cursor==null) return;
StringBuffer buffer= new StringBuffer();
while (cursor.moveToNext()){
String name=cursor.getString(1);
String email= cursor.getString(3);
}
new AlertDialog.Builder(this).setMessage(buffer.toString()).setPositiveButton("OK",null).create().show();
}
public void clickSelectByName(View view) {
String name= etName.getText().toString();
Cursor cursor=db.rawQuery("SELECT name, age FROM "+tableName+" WHERE name=?",new String[]{name});
if(cursor==null) return;
//참고: 총 레코드 수(줄,행(row)수)
int rowNum= cursor.getCount(); //데이터의 행의 수
StringBuffer buffer= new StringBuffer();
while(cursor.moveToNext()){
String na=cursor.getString(0);
Toast.makeText(this, buffer.toString(), Toast.LENGTH_SHORT).show();
}
}
public void clickUpdate(View view) {
String name = etName.getText().toString();
}
public void clickDelete(View view) {
String name =etName.getText().toString();
db.execSQL("DELETE FROM "+tableName+" WHERE name=?",new String[]{name});
}
}
|
반응형
댓글